Feedback
Feedback endpoint
feedback
is an additional endpoint of Mindee API created to send feedbacks on a given prediction.
Note
The feedback endpoint is available on all Document API on demand.
The objective is to enable computation of performances on the user production flow per field. Performances will be generated by Mindee first and shared on a regular basis.
The process is the following:
- User sends a document through a POST request on the predict route.
- User receives in the response the prediction and the document ID
- Prediction is displayed and validated on the user’s product
- User sends a PUT or PATCH request to the feedback using the document ID and the payload (including validated values for all the used fields).
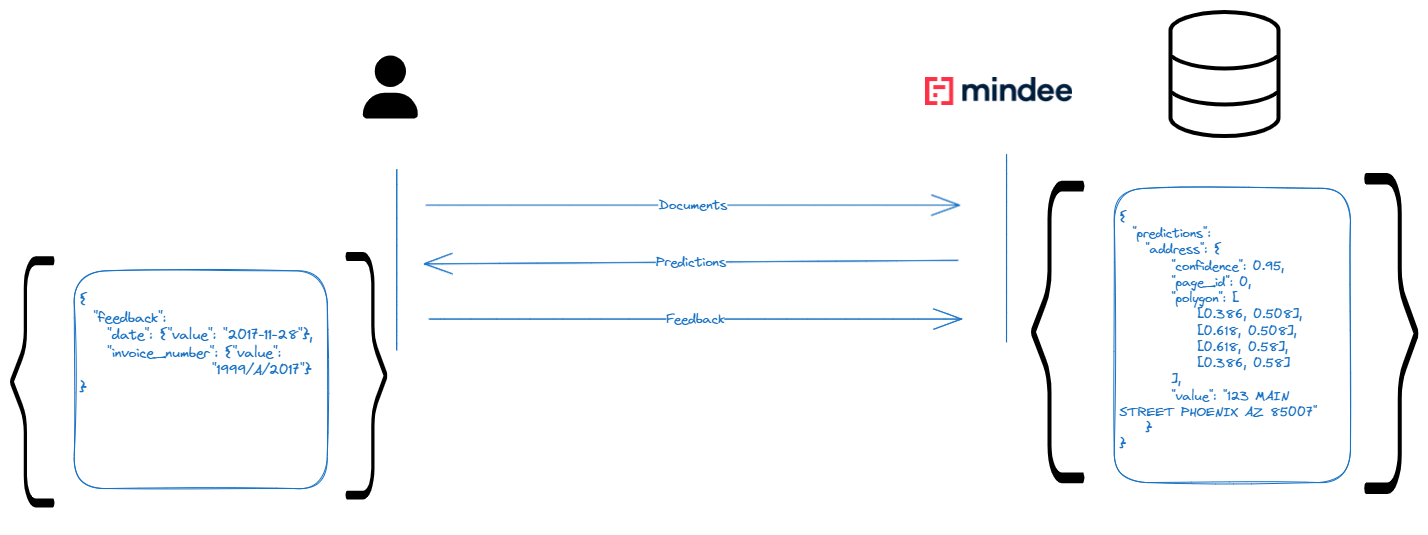
For example, on the Invoices API, in the case where you have the following fields validated on your interface by your end users:
- supplier_name
- total_amount
- due_date
- taxes
All 4 fields will need to be added in the payload of the feedback endpoint:
{
"feedback": {
"date": {"value": "2018-08-03"},
"supplier_name": {"value": "Metro"},
"taxes": [
{
"rate": 5,
"value": 40
},
{
"rate": 20,
"value": 750
}
],
"total_amount": {"value": 760.26}
}
}
How to use the feedback endpoint?
URL
To send feedback on a given prediction, use the following URL v1/documents/<document_id>/feedback
 where:
<document_id>
 refers to the document_id of the prediction itself
Methods available:
PUT: requires at least one field
PUT https://api.mindee.net/v1/documents/<document_id>/feedback
PATCH: requires at least one field
PATCH https://api.mindee.net/v1/documents/<document_id>/feedback
Both routes will return a similar payload, the updated feedback for the target document.
Payload: The document_id links the prediction, the feedback and the document
The feedback endpoint needs the full list of validated values from fields you are using from the prediction as a payload. You do not need to send back fields you are not using in your application.
import requests
# Step 1 : get prediction
url = "https://api.mindee.net/v1/products/<account_name>/<api_name>/<api_version>/predict"
with open("/path/to/my/file", "rb") as myfile:
files = {"document": myfile}
headers = {"Authorization": "Token my-api-key-here"}
response = requests.post(url, files=files, headers=headers)
# get document_id from the prediction
json_response = response.json()
document_id = json_response["document"]["id"]
#....
# values being validated by the end user
# ...
# Step 2: send feedback
url = f"https://api.mindee.net/v1/documents/{document_id}/feedback"
# Send all the validated values you are using in your application.
payload = json.dumps({
"feedback": {
"date": {"value": "2018-08-03"},
"supplier_name": {"value": "Metro"},
"taxes": [
{
"rate": 5,
"value": 40
},
{
"rate": 20,
"value": 750
}
],
"total_amount": {"value": 760.26}
}
})
headers = {
'Authorization': "Token <my-api-key-here>",
'Content-Type': 'application/json'
}
response = requests.put(url, headers=headers, data=payload)
print(response.text)
Error management
Error code | Description |
---|---|
400 | Payload error |
401 | Token error |
403 | Valid subscription but feedback endpoint is not enabled. |
404 | Wrong document ID |
Questions?
  Join our Slack
Updated about 2 months ago