ID Card France OCR
Automatically extract data from french ID cards.
Mindee’s ID Card France OCR API uses deep learning to automatically, accurately, and instantaneously parse your ID card details. In under a second, the API extracts a set of data from your PDFs or photos of ID cards, including:
- Nationality
- Card Access Number
- Document Number
- Given Names
- Surname
- Alternate Name
- Date of Birth
- Place of Birth
- Gender
- Expiry Date
- Image Orientation
- MRZ Line 1
- MRZ Line 2
- MRZ Line 3
- Date of Issue
- Issuing Authority
- Document Type
- Document Sides
The ID Card France OCR API supports the new and old format for all people in France. The ID cards of other nationalities and states are not supported with this model.
Set up the API
Before making any API calls, you need to have created your API key.
- You'll need a french ID card. You can use one of the sample documents provided below.
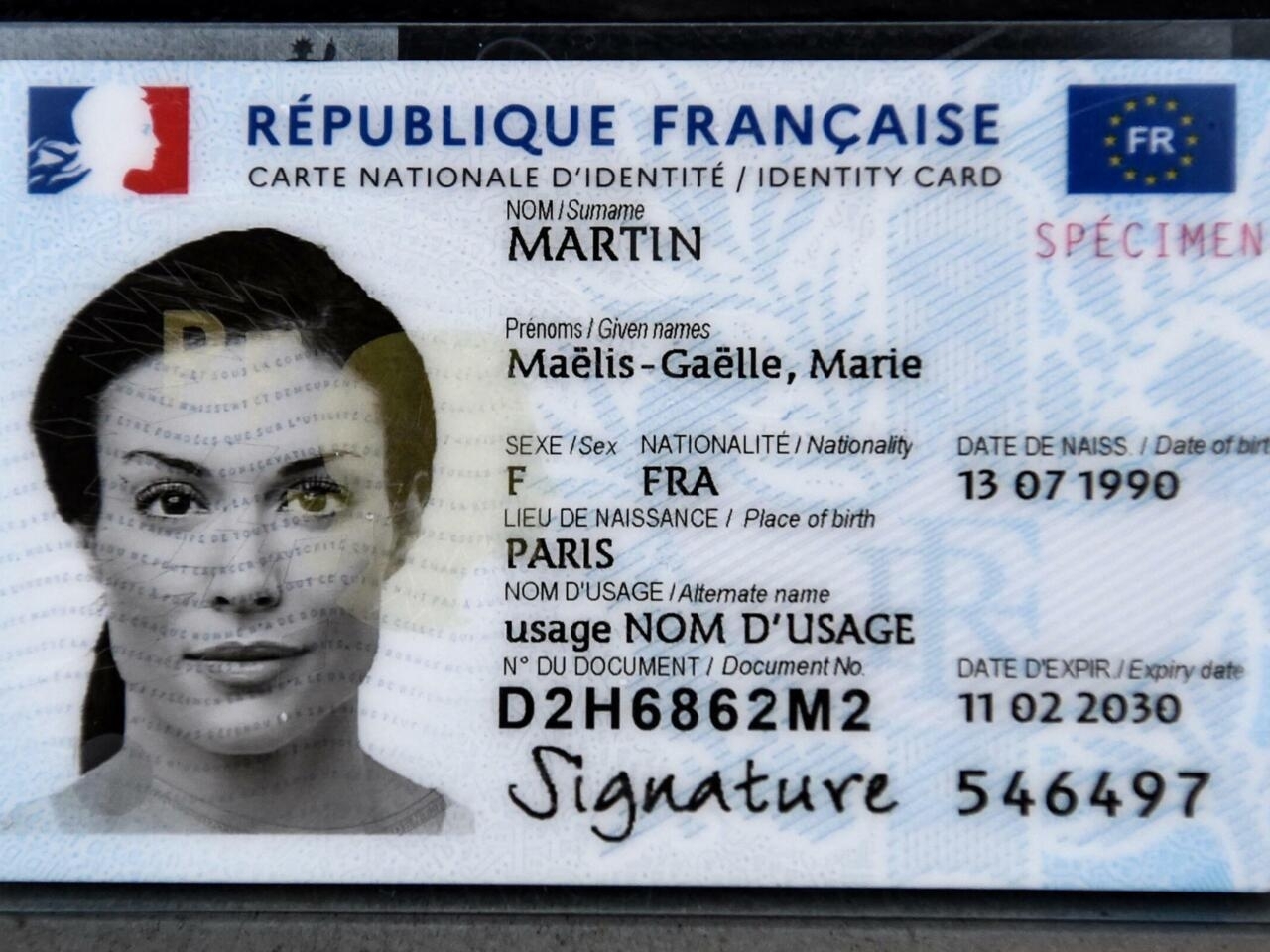
- Access your Receipt API by clicking on the Identity Card - FR card in the Document Catalog
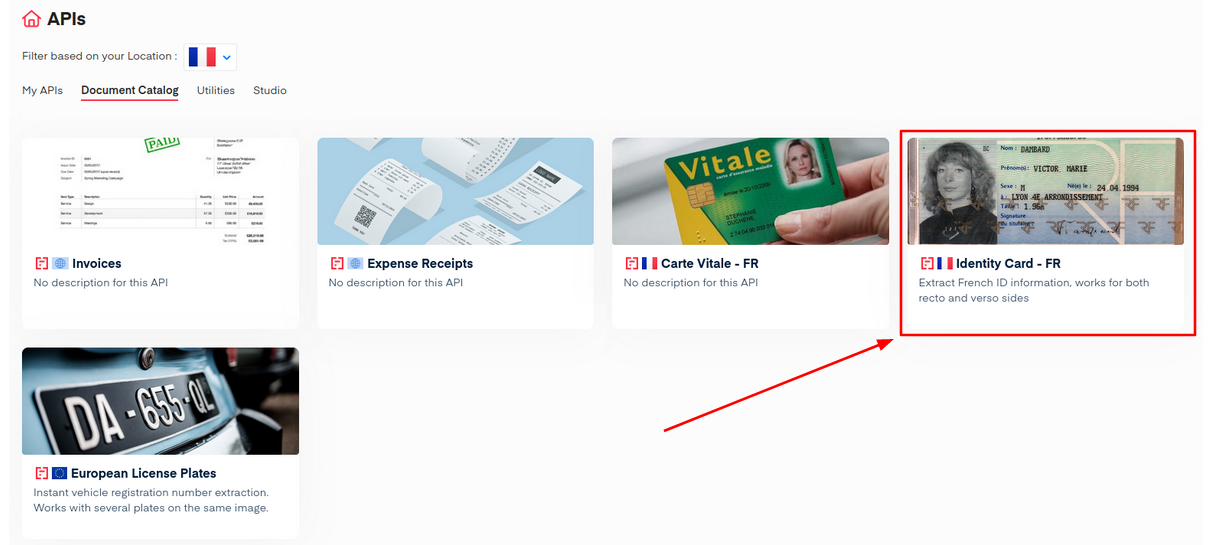
- From the left navigation, go to documentation > API Reference, you'll find sample code in popular languages and command line.
curl -X POST \\
https://api.mindee.net/v2/products/mindee/idcard_fr/v2/predict \\
-H 'Authorization: Token my-api-key-here' \\
-H 'content-type: multipart/form-data' \\
-F document=@/path/to/your/file.png
from mindee import Client, documents
# Init a new client
mindee_client = Client(api_key="my-api-key-here")
# Load a file from disk
input_doc = mindee_client.doc_from_path("/path/to/the/file.ext")
# Parse the Carte Nationale d'Identité by passing the appropriate type
result = input_doc.parse(documents.fr.TypeIdCardV1)
# Print a brief summary of the parsed data
print(result.document)
const mindee = require("mindee");
// for TS or modules:
// import * as mindee from "mindee";
// Init a new client
const mindeeClient = new mindee.Client({ apiKey: "my-api-key-here" });
// Load a file from disk and parse it
const apiResponse = mindeeClient
.docFromPath("/path/to/the/file.ext")
.parse(mindee.fr.IdCardV2);
// Handle the response Promise
apiResponse.then((resp) => {
// The `document` property can be undefined:
// * TypeScript will not compile without this guard clause
// (or consider using the '?' notation)
// * JavaScript will be very happy to produce subtle bugs
// without this guard clause
if (resp.document === undefined) return;
// print the full object
// console.log(resp.document);
// print a string summary
console.log(resp.document.toString());
});
require 'mindee'
# Init a new client
mindee_client = Mindee::Client.new(api_key: 'my-api-key-here')
# Load a file from disk
input_source = mindee_client.source_from_path('/path/to/the/file.ext')
# Parse the file
result = mindee_client.parse(
input_source,
Mindee::Product::FR::IdCard::IdCardV2
)
# Print a full summary of the parsed data in RST format
puts result.document
# Print the document-level parsed data
# puts result.document.inference.prediction
<form onsubmit="mindeeSubmit(event)" >
<input type="file" id="my-file-input" name="file" />
<input type="submit" />
</form>
<script type="text/javascript">
const mindeeSubmit = (evt) => {
evt.preventDefault()
let myFileInput = document.getElementById('my-file-input');
let myFile = myFileInput.files[0]
if (!myFile) { return }
let data = new FormData();
data.append("document", myFile, myFile.name);
let xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.mindee.net/v2/products/mindee/idcard_fr/v2/predict");
xhr.setRequestHeader("Authorization", "Token my-api-key-here");
xhr.send(data);
}
</script>
using Mindee;
using Mindee.Input;
using Mindee.Product.Fr.IdCard;
string apiKey = "my-api-key-here";
string filePath = "/path/to/the/file.ext";
// Construct a new client
MindeeClient mindeeClient = new MindeeClient(apiKey);
// Load an input source as a path string
// Other input types can be used, as mentioned in the docs
var inputSource = new LocalInputSource(filePath);
// Call the API and parse the input
var response = await mindeeClient
.ParseAsync<IdCardV1>(inputSource);
// Print a summary of all the predictions
System.Console.WriteLine(response.Document.ToString());
// Print only the document-level predictions
// System.Console.WriteLine(response.Document.Inference.Prediction.ToString());
import com.mindee.MindeeClient;
import com.mindee.input.LocalInputSource;
import com.mindee.parsing.common.PredictResponse;
import com.mindee.product.fr.idcard.IdCardV1;
import java.io.File;
import java.io.IOException;
public class SimpleMindeeClient {
public static void main(String[] args) throws IOException {
String apiKey = "my-api-key-here";
String filePath = "/path/to/the/file.ext";
// Init a new client
MindeeClient mindeeClient = new MindeeClient(apiKey);
// Load a file from disk
LocalInputSource inputSource = new LocalInputSource(new File(filePath));
// Parse the file
PredictResponse<IdCardV1> response = mindeeClient.parse(
IdCardV1.class,
inputSource
);
// Print a summary of the response
System.out.println(response.toString());
// Print a summary of the predictions
// System.out.println(response.getDocument().toString());
// Print the document-level predictions
// System.out.println(response.getDocument().getInference().getPrediction().toString());
// Print the page-level predictions
// response.getDocument().getInference().getPages().forEach(
// page -> System.out.println(page.toString())
// );
}
}
<?php
// cURL install check
if (!function_exists('curl_init')) {
exit("cURL isn't installed for " . phpversion());
}
$API_KEY = 'my-api-key-here';
$FILE_PATH = '/path/to/my/file.pdf';
$MIME_TYPE = 'application/pdf'; // change according to the file type
$ACCOUNT = 'mindee';
$VERSION = '2.0';
$ENDPOINT = 'idcard_fr';
// Open a cURL session to send the document
$ch = curl_init();
// Setup headers
$headers = array(
"Authorization: Token $API_KEY"
);
// Add our file to the request
$data = array(
"document" => new CURLFile(
$FILE_PATH,
$MIME_TYPE,
substr($FILE_PATH, strrpos($FILE_PATH, "/") + 1)
)
);
// URL for a prediction
$url = "https://api.mindee.net/v2/products/$ACCOUNT/$ENDPOINT/v$VERSION/predict";
$options = array(
CURLOPT_URL => $url,
CURLOPT_HTTPHEADER => $headers,
CURLOPT_POSTFIELDS => $data,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_RETURNTRANSFER => true
);
// Set all options for the cURL request
curl_setopt_array(
$ch,
$options
);
// Execute the request & extract the query content into a variable
$json = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Store the response as an array to allow for easier manipulations
$result = json_decode($json, true);
// Print the content of the document as raw json
echo json_encode($result, JSON_PRETTY_PRINT);
- Replace my-api-key-here with your new API key, or use the "select an API key" feature and it will be filled automatically.
- Copy and paste the sample code of your desired choice in your application, code environment or terminal.
- Replace
/path/to/my/file
with the path to your receipt.
Always remember to replace your API key!
- Run your code. You will receive a JSON response with the receipt details.
API Response
Here is the full JSON response you get when you call the API:
{
"api_request": {
"error": {},
"resources": [
"document"
],
"status": "success",
"status_code": 201,
"url": "https://api.mindee.net/v2/products/mindee/idcard_fr/v2/predict"
},
"document": {
"id": "07f38b63-189e-49d4-a07b-bdc0f49a8135",
"name": "sample_receipt.jpg",
"n_pages": 1,
"is_rotation_applied": true,
"inference": {
"started_at": "2023,-05-06T16:37:28",
"finished_at": "2023-05-06T16:37:29",
"processing_time": 1.755,
"pages": [
{
"id": 0,
"orientation": {"value": 0},
"prediction": { .. },
"extras": {}
}
],
"prediction": { .. },
"extras": {}
}
}
}
You can find the prediction within the prediction
key found in two locations:
- In
document > inference > prediction
for document-level predictions: it contains the different fields extracted at the document level, meaning that for multi-pages PDFs, we reconstruct a single receipt object using all the pages. - In
document > inference > pages[ ] > prediction
for page-level predictions: it gives the prediction for each page independently. With images, there is only one element on this array, but with PDFs, you can find the extracted data for each PDF page.
Each predicted field may contain one or several values:
- a
confidence
score - a
polygon
highlighting the information location - a
page_id
where the information was found (document level only)
{
"extras": {},
"finished_at": "2023-08-01T08:03:02.691624",
"is_rotation_applied": true,
"pages": [
{
"extras": {},
"id": 0,
"orientation": {"value": 0},
"prediction": {
"alternate_name": {
"confidence": 0.51,
"polygon": [
[0.393, 0.648],
[0.722, 0.648],
[0.722, 0.682],
[0.393, 0.682]
],
"value": "usage NOM D'USAGE"
},
"authority": {
"confidence": 0,
"polygon": [],
"value": null
},
"birth_date": {
"confidence": 0.99,
"polygon": [
[0.749, 0.491],
[0.929, 0.491],
[0.929, 0.525],
[0.749, 0.525]
],
"value": "1990-07-13"
},
"birth_place": {
"confidence": 0.99,
"polygon": [
[0.394, 0.562],
[0.485, 0.562],
[0.485, 0.598],
[0.394, 0.598]
],
"value": "PARIS"
},
"card_access_number": {
"confidence": 0.99,
"polygon": [
[0.745, 0.805],
[0.959, 0.805],
[0.959, 0.857],
[0.745, 0.857]
],
"value": "546497"
},
"document_number": {
"confidence": 0.99,
"polygon": [
[0.385, 0.718],
[0.687, 0.718],
[0.687, 0.768],
[0.385, 0.768]
],
"value": "D2H6862M2"
},
"document_side": {
"confidence": 1,
"value": "RECTO"
},
"document_type": {
"confidence": 1,
"value": "NEW"
},
"expiry_date": {
"confidence": 0.88,
"polygon": [
[0.749, 0.724],
[0.933, 0.724],
[0.933, 0.759],
[0.749, 0.759]
],
"value": "2030-02-11"
},
"gender": {
"confidence": 0.99,
"polygon": [
[0.396, 0.487],
[0.417, 0.487],
[0.417, 0.52],
[0.396, 0.52]
],
"value": "F"
},
"given_names": [
{
"confidence": 0.74,
"polygon": [
[
0.398,
0.362
],
[
0.62,
0.362
],
[
0.62,
0.401
],
[
0.398,
0.401
]
],
"value": "Maëlis-gaëlle"
},
{
"confidence": 0.99,
"polygon": [
[
0.635,
0.363
],
[
0.727,
0.363
],
[
0.727,
0.398
],
[
0.635,
0.398
]
],
"value": "Marie"
}
],
"issue_date": {
"confidence": 0,
"polygon": [],
"value": null
},
"mrz1": {
"confidence": 0,
"polygon": [],
"value": null
},
"mrz2": {
"confidence": 0,
"polygon": [],
"value": null
},
"mrz3": {
"confidence": 0,
"polygon": [],
"value": null
},
"nationality": {
"confidence": 0.99,
"polygon": [
[0.501, 0.487],
[0.566, 0.487],
[0.566, 0.522],
[0.501, 0.522]
],
"value": "FRA"
},
"orientation": {
"confidence": 0.99,
"degrees": 0
},
"surname": {
"confidence": 0.84,
"polygon": [
[0.396, 0.236],
[0.558, 0.236],
[0.558, 0.275],
[0.396, 0.275]
],
"value": "MARTIN"
}
}
}
],
"prediction": {
"alternate_name": {
"confidence": 0.51,
"page_id": 0,
"polygon": [
[0.393, 0.648],
[0.722, 0.648],
[0.722, 0.682],
[0.393, 0.682]
],
"value": "usage NOM D'USAGE"
},
"authority": {
"confidence": 0,
"page_id": null,
"polygon": [],
"value": null
},
"birth_date": {
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.749, 0.491],
[0.929, 0.491],
[0.929, 0.525],
[0.749, 0.525]
],
"value": "1990-07-13"
},
"birth_place": {
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.394, 0.562],
[0.485, 0.562],
[0.485, 0.598],
[0.394, 0.598]
],
"value": "PARIS"
},
"card_access_number": {
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.745, 0.805],
[0.959, 0.805],
[0.959, 0.857],
[0.745, 0.857]
],
"value": "546497"
},
"document_number": {
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.385, 0.718],
[0.687, 0.718],
[0.687, 0.768],
[0.385, 0.768]
],
"value": "D2H6862M2"
},
"document_side": {
"confidence": 1,
"value": "RECTO"
},
"document_type": {
"confidence": 1,
"value": "NEW"
},
"expiry_date": {
"confidence": 0.88,
"page_id": 0,
"polygon": [
[0.749, 0.724],
[0.933, 0.724],
[0.933, 0.759],
[0.749, 0.759]
],
"value": "2030-02-11"
},
"gender": {
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.396, 0.487],
[0.417, 0.487],
[0.417, 0.52],
[0.396, 0.52]
],
"value": "F"
},
"given_names": [
{
"confidence": 0.74,
"page_id": 0,
"polygon": [
[0.398, 0.362],
[0.62, 0.362],
[0.62, 0.401],
[0.398, 0.401]
],
"value": "Maëlis-gaëlle"
},
{
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.635, 0.363],
[0.727, 0.363],
[0.727, 0.398],
[0.635, 0.398]
],
"value": "Marie"
}
],
"issue_date": {
"confidence": 0,
"page_id": null,
"polygon": [],
"value": null
},
"mrz1": {
"confidence": 0,
"page_id": null,
"polygon": [],
"value": null
},
"mrz2": {
"confidence": 0,
"page_id": null,
"polygon": [],
"value": null
},
"mrz3": {
"confidence": 0,
"page_id": null,
"polygon": [],
"value": null
},
"nationality": {
"confidence": 0.99,
"page_id": 0,
"polygon": [
[0.501, 0.487],
[0.566, 0.487],
[0.566, 0.522],
[0.501, 0.522]
],
"value": "FRA"
},
"surname": {
"confidence": 0.84,
"page_id": 0,
"polygon": [
[0.396, 0.236],
[0.558, 0.236],
[0.558, 0.275],
[0.396, 0.275]
],
"value": "MARTIN"
}
},
"processing_time": 1.272,
"product": {
"features": [
"nationality",
"card_access_number",
"document_number",
"given_names",
"surname",
"alternate_name",
"birth_date",
"birth_place",
"gender",
"expiry_date",
"orientation",
"mrz1",
"mrz2",
"mrz3",
"issue_date",
"authority",
"document_type",
"document_side"
],
"name": "mindee/idcard_fr",
"type": "standard",
"version": "2.0"
},
"started_at": "2023-08-01T08:03:01.419255"
}
Extracted ID card data
Depending on the field type specified, additional attributes can be extracted from the ID card object. Using the above ID card example the following are the basic fields that can be extracted.
- Nationality
- Card Access Number
- Document Number
- Given Names
- Surname
- Alternate Name
- Date Of Birth
- Place Of Birth
- Gender
- Expiry Date
- Image Orientation
- MRZ Line 1
- MRZ Line 2
- MRZ Line 3
- Date Of Issue
- Issuing Authority
- Document Type
- Document Sides
Nationality
- nationality: The nationality is classified, issued in a three-letter code following the ISO 3166-1 format.
{
"nationality": {
"confidence": 0.99,
"polygon": [
[0.501, 0.487],
[0.566, 0.487],
[0.566, 0.522],
[0.501, 0.522]
],
"value": "FRA"
}
}
Card Access Number
- card_access_number: Unique CAN of the document. There is no associated field name for this number on the card itself.
{
"card_access_number": {
"confidence": 0.99,
"polygon": [
[0.745, 0.805],
[0.959, 0.805],
[0.959, 0.857],
[0.745, 0.857]
],
"value": "546497"
}
}
Document Number
- document_number: The unique ID number of the document which is likewise titled.
{
"document_number": {
"confidence": 0.99,
"polygon": [
[0.385, 0.718],
[0.687, 0.718],
[0.687, 0.768],
[0.385, 0.768]
],
"value": "D2H6862M2"
}
}
Given Names
- given_names: The given Name(s) of the card holder. If there are several names, they are all output as in the following example:
{
"given_names": [
{
"confidence": 0.74,
"polygon": [
[
0.398,
0.362
],
[
0.62,
0.362
],
[
0.62,
0.401
],
[
0.398,
0.401
]
],
"value": "Maëlis-gaëlle"
},
{
"confidence": 0.99,
"polygon": [
[
0.635,
0.363
],
[
0.727,
0.363
],
[
0.727,
0.398
],
[
0.635,
0.398
]
],
"value": "Marie"
}
}
Surname
- surname: The last name of the document holder. f there are several surnames, all surnames are output together.
{
"surname": {
"confidence": 0.84,
"polygon": [
[0.396, 0.236],
[0.558, 0.236],
[0.558, 0.275],
[0.396, 0.275]
],
"value": "MARTIN"
}
}
Alternate Name
- alternate_name: The alternate name of the document holder. The same rules apply as for the other names.
{
"alternate_name": {
"confidence": 0.51,
"polygon": [
[0.393, 0.648],
[0.722, 0.648],
[0.722, 0.682],
[0.393, 0.682]
],
"value": "usage NOM D'USAGE"
}
}
Date Of Birth
- date_of_birth: The ISO formatted date of birth of the document holder in the ISO 8601 format.
{
"birth_date": {
"confidence": 0.99,
"polygon": [
[0.749, 0.491],
[0.929, 0.491],
[0.929, 0.525],
[0.749, 0.525]
],
"value": "1990-07-13"
}
}
Place Of Birth
- place_of_birth: The Place of Birth of the Document holder.
{
"birth_place": {
"confidence": 0.99,
"polygon": [
[0.394, 0.562],
[0.485, 0.562],
[0.485, 0.598],
[0.394, 0.598]
],
"value": "PARIS"
}
}
Gender
- gender: Output in a code consisting of one letter representing the gender of the document owner.
{
"gender": {
"confidence": 0.99,
"polygon": [
[0.396, 0.487],
[0.417, 0.487],
[0.417, 0.52],
[0.396, 0.52]
],
"value": "F"
}
}
Expiry Date
- expiry_date: The ISO formatted date of expiry of the document in the ISO 8601 format.
{
"expiry_date": {
"confidence": 0.88,
"polygon": [
[0.749, 0.724],
[0.933, 0.724],
[0.933, 0.759],
[0.749, 0.759]
],
"value": "2030-02-11"
}
}
Image Orientation
- image_orientation: Classification indicating the possible rotation of the image. Possible values are:
- 0
- 90
- 270
Images with a rotation of 180 degrees are not supported.
{
"orientation": {
"confidence": 0.99,
"degrees": 0
}
}
MRZ Line 1
- mrz_line_1: The extraction of the Machine Readable Zone, first line.
{
"mrz1": {
"confidence": 0.98,
"polygon": [
[0.024, 0.694],
[0.968, 0.694],
[0.968, 0.747],
[0.024, 0.747]
],
"value": "IDFRAX4RTBPFW46<<<<<<<<<<<<<<<"
}
}
MRZ Line 2
- mrz_line_2: The extraction of the Machine Readable Zone, second line.
{
"mrz2": {
"confidence": 0.96,
"polygon": [
[0.024, 0.77],
[0.992, 0.77],
[0.992, 0.824],
[0.024, 0.824]
],
"value": "9007138F3002119FRA<<<<<<<<<<<6"
}
}
MRZ Line 3
- mrz_line_3: The extraction of the Machine Readable Zone, third line.
{
"mrz3": {
"confidence": 0.91,
"polygon": [
[0.024, 0.848],
[0.997, 0.848],
[0.997, 0.902],
[0.024, 0.902]
],
"value": "MARTIN<<MAELYS<GAELLE<MARIE<<<"
}
}
Date Of Issue
- date_of_issue: The ISO formatted date of issue of the document in the ISO 8601 format.
{
"issue_date": {
"confidence": 0.99,
"polygon": [
[0.417, 0.093],
[0.584, 0.093],
[0.584, 0.131],
[0.417, 0.131]
],
"value": "2020-02-12"
}
}
Issuing Authority
- issuing_authority: The responsible authority for issuing the document.
{
"authority": {
"confidence": 0,
"polygon": [],
"value": null
}
}
Document Type
- document_type: Classification indicating which version of the ID card it is.
- NEW - the issued version from 2021
- OLD - the issued version from 1988 - 2021
{
"document_type": {
"confidence": 1,
"value": "NEW"
}
}
Document Sides
- document_sides: Classification indicating which version of the ID card it is.
- RECTO - the front side
- VERSO - the back side
{
"document_side": {
"confidence": 1,
"value": "RECTO"
}
}
Questions?
Join our Slack
Updated 5 months ago